I have created this simple WordPress plugin (Jfa Social Media Post) that will allow you to retrieve a specific Instagram post and consume it through the WP API REST.
This is very practical if you have built the front of your WordPress site with a modern javascript framework like React or Vue. Retrieve the data from the Instagram post via API and inject it into your design.
Getting Started
In your WordPress menu go to Plugins > Add New
and search: Jfa Social Media Post
install and active it.
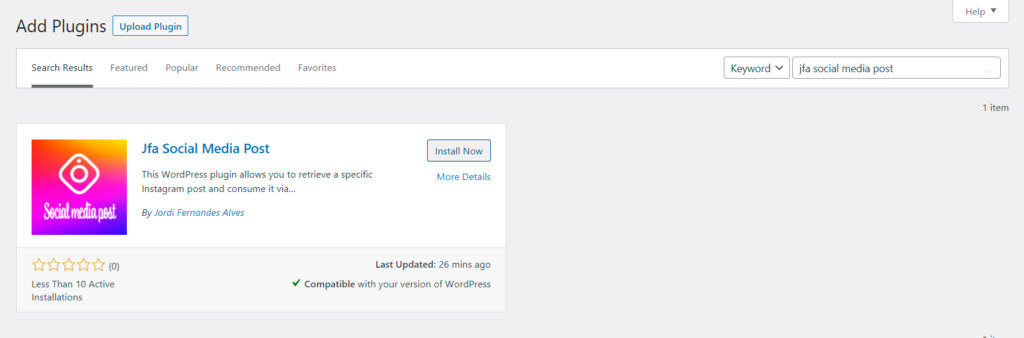
Enter https://instant-tokens.com and create the API URL
of your Instagram account and paste it in config.
Then in Post displayed
paste the URL of the Instagram post of your account that you want to show and click the Save changes
button. You can change the URL of the post whenever you want.
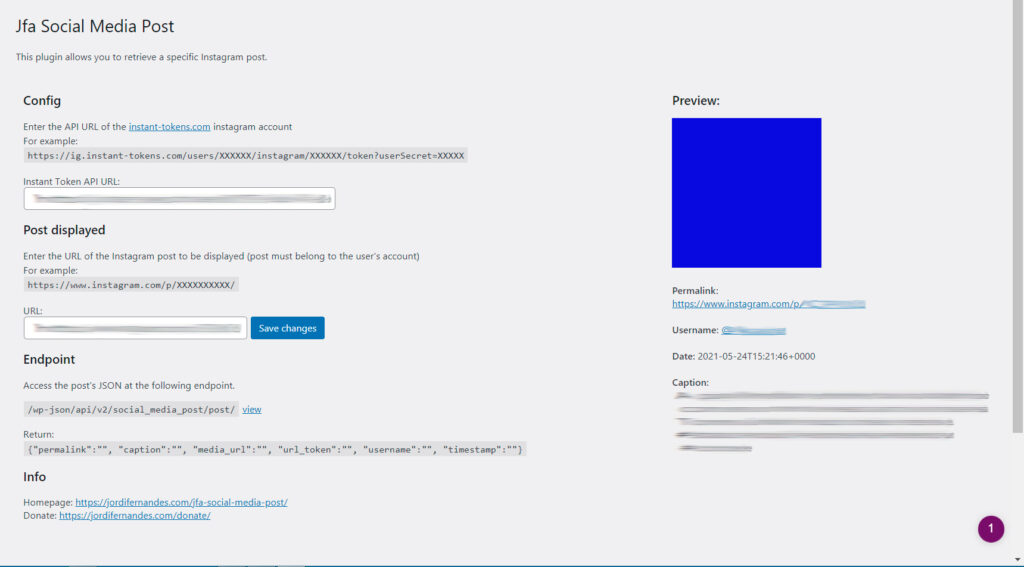
Endpoint
Retrieve the post data via the following REST API endpoint:
GET /wp-json/api/v2/social_media_post/post/
JSON Return
{ "permalink": "https://www.instagram.com/p/XXXXXXXXXXXX/", "caption": "Caption text", "media_url": "https://scontent-ams4-1.cdninstagram.com/v/XXX/XXX.jpg?_nc_cat=X&ccb=1-5&_nc_sid=XX&_nc_ohc=XXX&_nc_ht=scontent-ams4-1.cdninstagram.com&edm=XXX", "url_token": "https://ig.instant-tokens.com/users/XXXXXX/instagram/XXXX/token.js?userSecret=XXXX", "username": "XXXXXXXX", "timestamp": "2020-12-14T20:12:36+0000" }
Front examples
Front example with JQuery
<h3>Preview:</h3> <img class="instagram_post_media_url" src=""> <p> <b>Permalink: </b><a class="instagram_post_permalink" href="" target="_blank"></a><br> <b>Username: </b><a class="instagram_post_username" href="" target="_blank"></a><br> <b>Date: </b><span class="instagram_post_timestamp"></span><br> <b>Caption: </b><span class="instagram_post_caption"></span> </p> <script> jQuery(document).ready(function() { jQuery.get('/wp-json/api/v2/social_media_post/post/', function(data) { jQuery('.instagram_post_media_url').attr('src', data.media_url); jQuery('.instagram_post_permalink').attr('href', data.permalink); jQuery('.instagram_post_permalink').html(data.permalink); jQuery('.instagram_post_username').attr('href', 'https://www.instagram.com' + data.username); jQuery('.instagram_post_username').html('@' + data.username); jQuery('.instagram_post_timestamp').html(data.timestamp); jQuery('.instagram_post_caption').html(data.caption); }); }); </script>
Front example with modern Js (vue, react, angular…)
const response = await fetch('/wp-json/api/v2/social_media_post/post/'); const instagramPost = await response.json(); console.log('media_url', instagramPost.media_url); console.log('permalink', instagramPost.permalink); console.log('username', instagramPost.username); console.log('timestamp', instagramPost.timestamp); console.log('caption', instagramPost.caption);